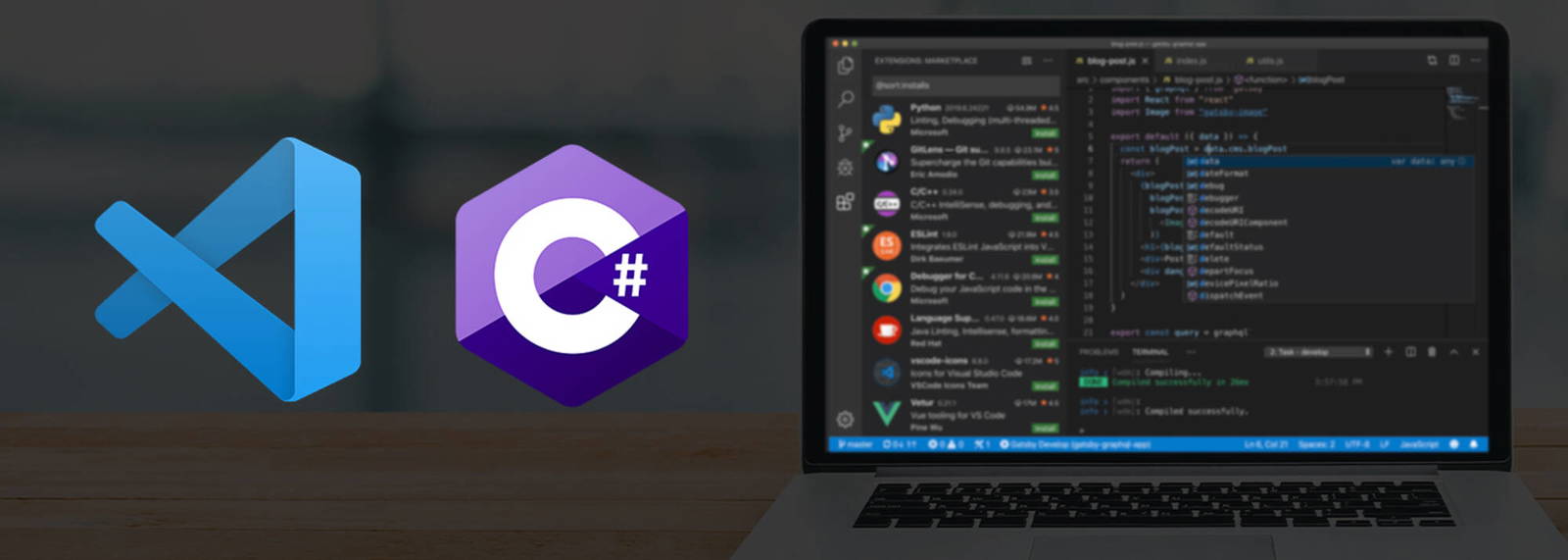
使用.net进行Algorand开发系列教程之ASA的创建及管理
本教程将讲解如何使用 C# SDK 完成 ASA 的创建和管理。
本教程的配套视频请查看:https://www.bilibili.com/video/BV1pK4y1f7rQ
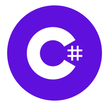
需要的工具和环境
背景
ASA 的全称是 Algorand Standard Asset,用户和开发者可以使用 ASA 在 Algorand 上开发实现上各种资产类型。ASA的应用场景特别丰富,如货币、稳定币和功能代币等资产。这里我们就再介绍如何使用.net SDK来进行ASA的相关操作。
本系列教程会有两节讲解ASA的相关操作,第一节(也就是本教程)主要介绍ASA的创建、修改相关信息及销毁。第二节(也就是本系列教程的第四节)主要介绍ASA的激活(Opt-In)、转账、撤销及冻结操作。
步骤
创建账户
我们使用三个账户来演示ASA的操作,这三个帐户在下面所有的操作中都会用到。注意:这三个账号只用于演示,在实际使用时永远不要直接将助记词放在代码中。
string ALGOD_API_ADDR = "ALGOD_API_ADDR"; //在此处添加ALGOD TESTNET API地址
string ALGOD_API_TOKEN = "ALGOD_API_TOKEN"; //在此处添加ALGOD API KEY
AlgodApi algodApiInstance = new AlgodApi(ALGOD_API_ADDR, ALGOD_API_TOKEN);
// 这三个账号只用于演示,在实际使用时永远不要直接将助记词放在代码中
string account1_mnemonic = "portion never forward pill lunch organ biology"
+ " weird catch curve isolate plug innocent skin grunt"
+ " bounce clown mercy hole eagle soul chunk type absorb trim";
string account2_mnemonic = "place blouse sad pigeon wing warrior wild script"
+ " problem team blouse camp soldier breeze twist mother"
+ " vanish public glass code arrow execute convince ability"
+ " there";
string account3_mnemonic = "image travel claw climb bottom spot path roast "
+ "century also task cherry address curious save item "
+ "clean theme amateur loyal apart hybrid steak about blanket";
Account acct1 = new Account(account1_mnemonic);
Account acct2 = new Account(account2_mnemonic);
Account acct3 = new Account(account3_mnemonic);
// 获取algorand网络的基本信息
var transParams = algodApiInstance.TransactionParams();
创建 ASA
创建ASA是故事的开始。在创建ASA的时候有5种角色:manager/reserve/freeze/clawback/sender.每种地址的意义请参照https://developer.algorand.org/docs/features/asa/.sender是无法设置与改变的,为创建资产这个操作的执行账号。默认情况下(即manager/reserve/freeze/clawback都没有设置的情况下)manager/reserve/freeze/clawback账号都是sender。如果设置了manager,其他没有设置的地址reserve/freeze/clawback都会是manager。
示例代码如下:
// Create the Asset(创建ASA)
// Total number of this asset available for circulation
var ap = new AssetParams(creator: acct1.Address.ToString(), assetname: "latikum22",
unitname: "LAT", defaultfrozen: false, total: 10000,
url: "http://this.test.com", metadatahash: Convert.ToBase64String(
Encoding.ASCII.GetBytes("16efaa3924a6fd9d3a4880099a4ac65d")))
{
// 每种地址的意义请参照https://developer.algorand.org/docs/features/asa/
// 默认情况下manager/reserve/freeze/clawback账号都是sender
// 如果设置了manager,其他没有设置的地址reserve/freeze/clawback都会是manager
Managerkey = acct1.Address.ToString(),
Clawbackaddr = acct2.Address.ToString(),
Freezeaddr = acct1.Address.ToString(),
Reserveaddr = acct1.Address.ToString()
};
var tx = Utils.GetCreateAssetTransaction(ap, transParams, "asset tx message");
// Sign the Transaction by sender
SignedTransaction signedTx = acct1.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
ulong? assetID = 0;
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
// Now that the transaction is confirmed we can get the assetID
Algorand.Algod.Client.Model.Transaction ptx = algodApiInstance.PendingTransactionInformation(id.TxId);
assetID = ptx.Txresults.Createdasset;
}
catch (Exception e)
{
Console.WriteLine(e.StackTrace);
return;
}
Console.WriteLine("AssetID = " + assetID);
// 现在ASA已经创建
这段代码与前两节的代码不同的是使用了大量的Utils函数。如 Utils.GetCreateAssetTransaction
/ Utils.SubmitTransaction
/ Utils.WaitTransactionToComplete
。这些函数的使用使代码的逻辑更加清晰,可读性大大增加。其实其效果和第(一)、(二)节中的相同。
修改 ASA 信息
修改ASA信息必须由Manage Address来执行。
// 修改ASA的设置
// Next we will change the asset configuration
// First we update standard Transaction parameters
// To account for changes in the state of the blockchain
transParams = algodApiInstance.TransactionParams();
ap = algodApiInstance.AssetInformation((long?)assetID);
// 修改ASA设置必须由manager账号执行,在本例是中acct2
// Note in this transaction we are re-using the asset
// creation parameters and only changing the manager
// and transaction parameters like first and last round
// now update the manager to acct1
ap.Managerkey = acct2.Address.ToString();
tx = Utils.GetConfigAssetTransaction(acct1.Address, assetID, ap, transParams, "config trans");
// The transaction must be signed by the current manager account
// We are reusing the signedTx variable from the first transaction in the example
signedTx = acct1.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id.TxId);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
}
catch (Exception e)
{
//e.printStackTrace();
Console.WriteLine(e.Message);
return;
}
// Next we will list the newly created asset
// Get the asset information for the newly changed asset
ap = algodApiInstance.AssetInformation((long?)assetID);
//The manager should now be the same as the creator
Console.WriteLine(ap);
销毁 ASA
如果一种ASA不再使用,可以将其毁(谨慎使用,操作不可逆)。
ASA的销毁操作必须由资产的Manage Address来进行操作,而且在进行操作时所有资产必须回到Creator账号中,否则此操作会失败。
代码如下,更详细说明请参照代码中注释:
// 销毁资产
// 销毁资产前所有资产需要回到创建者账号中
// 销毁资产需要由Manage Addr进行操作
// First we update standard Transaction parameters
// To account for changes in the state of the blockchain
transParams = algodApiInstance.TransactionParams();
// Next we set asset xfer specific parameters
// The manager must sign and submit the transaction
// This is currently set to acct2
tx = Utils.GetDestroyAssetTransaction(acct2.Address, assetID, transParams, "destroy transaction");
// The transaction must be signed by the manager account
// We are reusing the signedTx variable from the first transaction in the example
signedTx = acct2.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
// We can now list the account information for acct1
// and see that the asset is no longer there
act = algodApiInstance.AccountInformation(acct1.Address.ToString());
}
catch (Exception e)
{
Console.WriteLine(e.Message);
return;
}
Console.WriteLine("You have successefully arrived the end of this test, please press and key to exist.");
Console.ReadKey();
总结
本文主要进行了ASA的基本介绍及ASA管理操作的执行。这些操作虽然不常用,但对ASA来说也至关重要。
本系列科程的所有代码都可以在https://github.com/RileyGe/algo-samples上找到。欢迎大家提Issues或Pull Request.