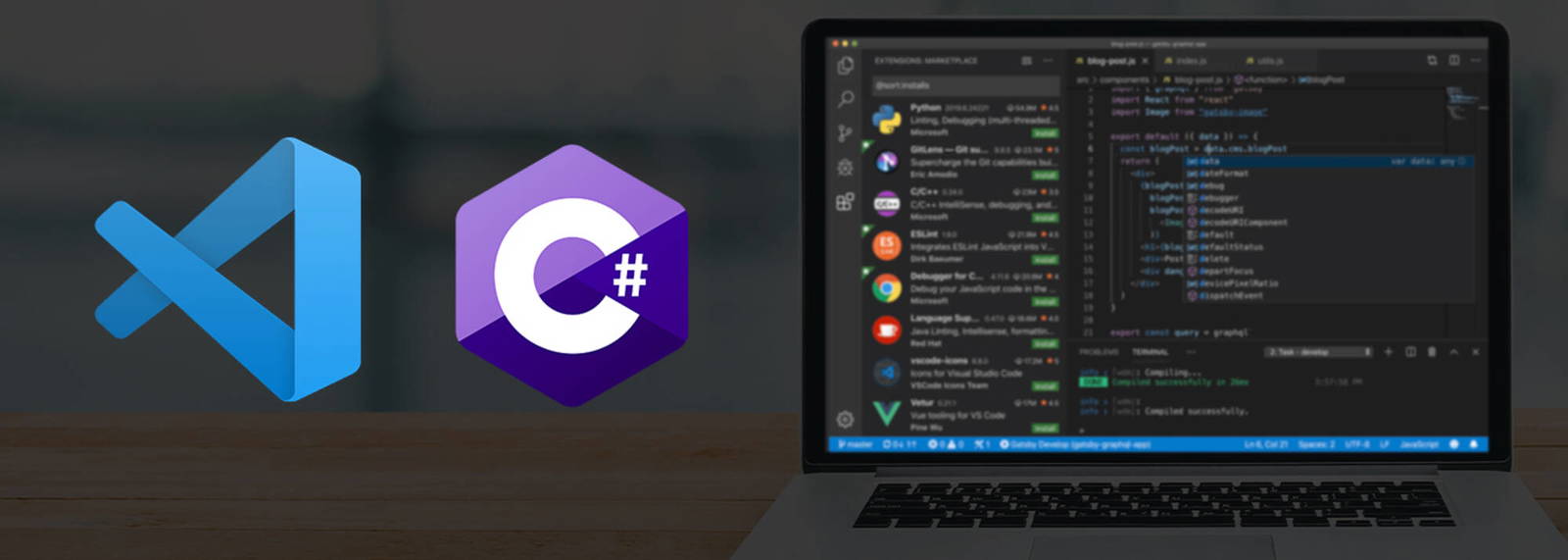
使用.net进行Algorand开发系列教程之ASA转账
本教程讲解如何使用 C# SDK 进行 ASA 的转账操作。
本教程的配套视频请查看:https://www.bilibili.com/video/BV1pK4y1f7rQ
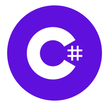
需要的工具和环境
背景
本教程与第三篇《使用.net进行Algorand开发系列教程之ASA的创建及管理》密切相关,甚至下面的操作需要使用第三篇中创建的ASA来进行操作。
步骤
激活(Opt-In)ASA
一个帐户在接收ASA之前,需要先激活(Opt-In),否则ASA的转账会失败。
本操作最好衔接在第三篇中的4.修改ASA信息之后,需要使用第三篇中创建的ASA的assetID来进行下述操作。
// 激活(Opt-In)某种ASA
// 如果你需要给其他用户转ASA,那么对方必须先激活
// 然后才能接收ASA
// First we update standard Transaction parameters
// To account for changes in the state of the blockchain
transParams = algodApiInstance.TransactionParams();
tx = Utils.GetActivateAssetTransaction(acct3.Address, assetID, transParams, "opt in transaction");
// The transaction must be signed by the current manager account
// We are reusing the signedTx variable from the first transaction in the example
signedTx = acct3.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
Algorand.Algod.Client.Model.Account act = null;
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id.TxId);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
// We can now list the account information for acct3
// and see that it can accept the new asseet
act = algodApiInstance.AccountInformation(acct3.Address.ToString());
Console.WriteLine(act);
}
catch (Exception e)
{
//e.printStackTrace();
Console.WriteLine(e.Message);
return;
}
ASA 转账
// ASA转账
// 激活后account3就可以接收ASA了
// 现在我们从acctout1向account3转账
// First we update standard Transaction parameters
// To account for changes in the state of the blockchain
transParams = algodApiInstance.TransactionParams();
// Next we set asset xfer specific parameters
// We set the assetCloseTo to null so we do not close the asset out
ulong assetAmount = 10;
tx = Utils.GetTransferAssetTransaction(acct1.Address, acct3.Address, assetID, assetAmount, transParams, null, "transfer message");
// The transaction must be signed by the sender account
// We are reusing the signedTx variable from the first transaction in the example
signedTx = acct1.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id.TxId);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
// We can now list the account information for acct3
// and see that it now has 5 of the new asset
act = algodApiInstance.AccountInformation(acct3.Address.ToString());
Console.WriteLine(act.GetHolding(assetID).Amount);
}
catch (Exception e)
{
//e.printStackTrace();
Console.WriteLine(e.Message);
return;
}
冻结 ASA
在某些特殊情况下,我们需要冻结ASA。这个操作必须由Freeze Address来进行。
// 冻结资产
// 如果freeze address当时没有设置,则无法冻结资产
// 此例中冻结account3中的资产
// 冻结事件须由freeze acount来发出,本例中为account1
// First we update standard Transaction parameters
// To account for changes in the state of the blockchain
transParams = algodApiInstance.TransactionParams();
// Next we set asset xfer specific parameters
// The sender should be freeze account acct2
// Theaccount to freeze should be set to acct3
tx = Utils.GetFreezeAssetTransaction(acct1.Address, acct3.Address, assetID, true, transParams, "freeze transaction");
// The transaction must be signed by the freeze account acct2
// We are reusing the signedTx variable from the first transaction in the example
signedTx = acct1.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id.TxId);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
// We can now list the account information for acct3
// and see that it now frozen
// Note--currently no getter method for frozen state
act = algodApiInstance.AccountInformation(acct3.Address.ToString());
Console.WriteLine(act.GetHolding(assetID).ToString());
}
catch (Exception e)
{
//e.printStackTrace();
Console.WriteLine(e.Message);
return;
}
撤回 ASA
在某些特殊情况下,如一个交易中卖方产生质量不合格等,我们可能需要撤回ASA。撤回操作至少需要两个账户来执行。
撤回操作的发起者为原转账操作的发起者。但只有原转账操作的发起者还不够,还需要ASA资产的Clawback账号进行签名。如果Manage Address将Clawback Address设为空的话,那么此资产将无法进行撤回操作。
// 撤回转账
// 撤加转账必须由clawbackaddress发起。
// 如果资产的manager将clawbackaddress设为空,则此操作不可执行
// 本例中会将10个资产从account3撤回到account1
// 此操作需要由clawbackaccount(account2)进行签名
// 此操作发送者为原操作的发起者(acct1)
// First we update standard Transaction parameters
// To account for changes in the state of the blockchain
transParams = algodApiInstance.TransactionParams();
// Next we set asset xfer specific parameters
assetAmount = 10;
tx = Utils.GetRevokeAssetTransaction(acct2.Address, acct3.Address, acct1.Address, assetID, assetAmount, transParams, "revoke transaction");
// The transaction must be signed by the clawback account
// We are reusing the signedTx variable from the first transaction in the example
signedTx = acct2.SignTransaction(tx);
// send the transaction to the network and
// wait for the transaction to be confirmed
try
{
TransactionID id = Utils.SubmitTransaction(algodApiInstance, signedTx);
Console.WriteLine("Transaction ID: " + id);
Console.WriteLine(Utils.WaitTransactionToComplete(algodApiInstance, id.TxId));
// We can now list the account information for acct3
// and see that it now has 0 of the new asset
act = algodApiInstance.AccountInformation(acct3.Address.ToString());
Console.WriteLine(act.GetHolding(assetID).Amount);
}
catch (Exception e)
{
Console.WriteLine(e.Message);
return;
}
总结
本文主要介绍了ASA的转账及相关操作。这些操作在ASA的使用中非常常用,特别是转账操作,是ASA所有操作中最核心的部分。
到目前为止,使用.net进行Algorand开发系列教程就告一段落了。本教程只进行了基本操作的执行方法,在此基础上大家可以开动脑筋,来创建更丰富、更有趣的应用。
本文所涉及的所有代码都发布在https://github.com/RileyGe/algo-samples项目中,希望大家能够积极的通过issue给本文提出意见与建议。