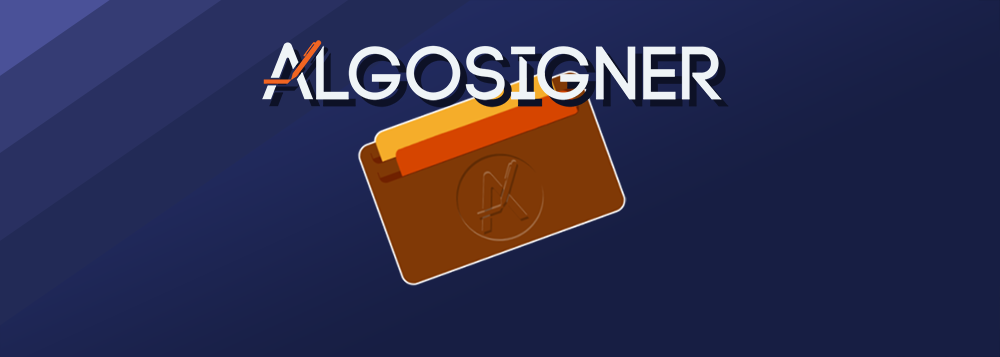
Adding Transaction Capabilities to a dApp Using AlgoSigner
AlgoSigner is an open-source wallet extension for Chrome that makes it easy to use Algorand-based applications on the web. In this tutorial, you will learn how to integrate AlgoSigner into your decentralized application so you can request to connect to a visitor’s wallet, send transactions for their signature, and transmit signed transactions to the Algorand TestNet or MainNet — all without needing to handle keys or secrets.
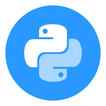
Requirements
You will need to install the AlgoSigner Chrome extension. Download AlgoSigner from the Chrome store or build from source.
If you’d liked to follow along using the sample dApp, you can view it here .
Background
PureStake recently released AlgoSigner , a browser-based blockchain wallet that makes it easy to use ALGOs with decentralized applications. AlgoSigner was funded by the Algorand Foundation’s grants program and is live in the Chrome store.
By integrating AlgoSigner into your dApps, you will be able to request to connect to a visitor’s wallet, send transactions for their signature, and transmit signed transactions to the Algorand TestNet or MainNet — all without needing to handle keys or secrets.
Let’s explore a little bit more about how AlgoSigner can be integrated into your solution. To help us in this journey, we’ve created a sample site, which you can visit here.
Steps
1. Connect to the Wallet
AlgoSigner injects a JavaScript library into every web page the user visits, which allows the site to interact with the extension. The dApp can use the injected library to connect to the user’s Wallet, discover account addresses it holds, query the network (make calls to algod
v2 or the algorand-indexer
) and request AlgoSigner to ask the user to sign a transaction initiated by the application. All methods of the injected library return a Promise that needs to be handled by the dApp.
In your application, you can implement the AlgoSigner.connect method to request access to the wallet for the dApp, and this may be rejected or approved by the user.
A demonstration code for this implementation in the example AlgoSigner dApp is shown at the right side of the button.
If the user grants access, in this example dApp, an empty object is returned as a response. Otherwise, the Promise will be rejected with an error message.
These cases need to be handled by the application being developed.
2. Query algod
to Get the Network Status
Developers can also leverage the AlgoSigner.algod method to make calls to a relay node in the network. This will return the current status, including the network and path to query.
In the following example, we will make a call to the algod
V2 API to query the status of the TestNet.
As you can see, the response provides all the information returned by the node.
AlgoSigner allows you to access any exposed endpoint and to configure those calls as needed. For example: POSTing a binary.
You can also use the AlgoSigner.indexer method to make a call to the algorand-indexer
. You can find more information on REST APIs in the following links:
algod
v2: https://developer.algorand.org/docs/reference/rest-apis/algod/v2/algorand-indexer
: https://developer.algorand.org/docs/reference/rest-apis/indexer/- The PureStake API Service allows you to interact with both APIs
3. Request Addresses in the Wallet
You can also request the addresses present in the wallet for a given network using the AlgoSigner.accounts method, by providing the network name (in this case either TestNet or MainNet).
The addresses available are returned as a JSON object.
4. Create and Sign a Transaction Using AlgoSigner
For this part, we’ll use the payment tab of our example dApp.
To be able to sign a transaction, we first need to create the transaction object. We can do this by querying algod
with the parameters path, in this example from the TestNet.
algod
will return an object. For this example, we’ve saved this object in the variable txParams. With it, we can construct the transaction object and pass it to the AlgoSigner.sign method. Note that, to populate the accounts from the Wallet, they need to be queried first.
Once the method is called, AlgoSigner will pop up, showing the user details regarding the transaction the dApp wants him or her to sign. After confirming, the transaction is signed by entering his or her password.
The response returned by the method is shown in the previous image, which includes the transaction ID, and the blob. For this example, we’ve stored the response as signedTx.
Note: The returned blob is not in the standard SDK format, but rather is base64 encoded. Due to limitations in Chrome internal messaging, AlgoSigner encodes the transaction blob in base64 strings. Helper functions are provided in the repository: Python and NodeJS
The last step is to send the signed transaction. To do this, we can use the AlgoSigner.send method, proving the network where we intend to send the transaction, and the transaction blob from the previous step.
We can use a block explorer, such as GoalSeeker, to check the transaction details.
5. Create an Algorand Standard Asset (ASA)
Assets are created by signing and sending a transaction as in our previous example. The main difference is that the transaction object is constructed in a different way, as we need to provide fields such as the asset name, the unit name, total issuance, and decimal points. More information regarding ASA can be found here. For this part, we’ll use the asset create tab of our example dApp.
After connecting to the AlgoSigner wallet to get the accounts and suggested transaction parameters, we can construct our transaction object and pass it to the AlgoSigner.sign method. As before, AlgoSigner will pop-up, so the user can confirm and sign the transaction requested by the application.
Then, as before, we can use the AlgoSigner.send method passing in the transaction blob. Once confirmed, we can use the transaction ID to view the details in GoalSeeker .
We can also take advantage of the AlgoSigner.indexer method to make queries to the algorand-indexer
API.
6. Opting in to Assets
In Algorand, to be able to hold an asset, you must first opt in (read more about this here). To do this, we can use the same methods described before, but then constructing a specific transaction object that allows our address to receive the asset we specify.
For this part, we’ll use the asset opt-in tab of our example dApp, and the asset we created in the previous step.
As in the previous case, we need to connect to the AlgoSigner wallet to get the accounts and the suggested transaction parameters. We can then create our transaction object by providing the asset index. We can get the index from within the AlgoSigner wallet, clicking the address from which the asset was created which, in this case, is 12220825.
Like before, we can pass the transaction blob to the AlgoSigner.send method, and verify the details of the transaction.
We can take advantage of the AlgoSigner.indexer method to check, for example, all assets created with that same name. For that, we need to construct the correct path (more information here).
The response, in this case, is an object displaying the assets with the same name (limited to 1 here, because of the value we introduced as limit), and all the asset-related information.
As seen from these examples, dApps can leverage AlgoSigner to easily and securely interact with Algorand. This only requires a few clicks from the user and minimal effort into implementing the AlgoSigner JavaScript library from the developer’s standpoint.
AlgoSigner is currently unable to sign Application Call Transactions. This limitation will be addressed in a future release. We welcome community contributions to the AlgoSigner GitHub repository.