
Create a Mobile App (Android/iOS) using Xamarin Forms
This tutorial teaches you how to:
- How to create Mobile Applications using the Xamarin Framework
- How to install and use the Algorand Package in your application
- Interactivity between your application and the Algorand network to create account addresses, fund account address, and also dispense funds into the account address.
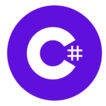
Requirements
- Visual Studio for Mac or Visual Studio Community 2019 for Windows
- .NET SDK Packages for Windows
Background
Creating Account Addresses should be easy. In this tutorial, we will learn how to generate account addresses on mobile devices. This example walks through the process of creating account addresses for use on the Algorand Blockchain Network.
This video demonstrates the entire process covered below:
Steps
1. Create New Xamarin Project in Visual Studio
Open Visual Studio, File | New (Search for Xamarin)
Figure 1-1
Search for Xamarin and Create a Xamarin Forms Application as shown in Figure 1-1
2. Install the Algorand Package
Insall the Algorand Package to your application. This can be done using the Package Manager Console with the following command:
“Install-Package Algorand”
Or using the NuGet Package Manager as shown in the image below:
Figure 1-2
3. Create an Account Class
To create an account class, navigate to the Models folder as shown in Figure 1-3 below
Figure 1-3
Add the following fields in your Account Class
Figure 1-4
4. Create a View Page
Figure 1-5
In the Views Folder, create a C# content Page called account address Page and follow along with the code snippet below:
using Algorand;
using Algorand.V2;
using MobileAlgorand.Models;
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Xamarin.Essentials;
using Xamarin.Forms;
namespace MobileAlgorand.Views
{
public class AccountPage : ContentPage
{
//Entry for Address
private Entry address;
//Entry for Balance
private Entry balance;
//Entry for Key
private Entry key;
//Storage fields for Accounnt Storeage for Restore
public string StorageAccountName1 = "Account 1";
public string StorageAccountName2 = "Account 2";
public string StorageAccountName3 = "Account 3";
public AccountPage()
{
//Page Title
this.Title = "Account Address Page";
StackLayout stackLayout = new StackLayout();
Button button = new Button();
button.Text = "Check Account Address Balance";
button.Clicked += Button_Clicked;
stackLayout.Children.Add(button);
//Address Text Entry Field
address = new Entry();
address.Placeholder = "Address Here..";
address.Keyboard = Keyboard.Default;
stackLayout.Children.Add(address);
//Account Balance Entry Field
balance = new Entry();
balance.Placeholder = "Balance Here..";
balance.Keyboard = Keyboard.Default;
stackLayout.Children.Add(balance);
//Account Key Address Field
key = new Entry();
key.Placeholder = "Key Here..";
key.Keyboard = Keyboard.Default;
stackLayout.Children.Add(key);
//Button to create an account address and mnemonic key
Button button2 = new Button();
button2.Text = "Generate Account Address";
button2.Clicked += Button2_Clicked;
stackLayout.Children.Add(button2);
Content = stackLayout;
}
//Button Event Handler to Check Account Balance
private async void Button_Clicked(object sender, EventArgs e)
{
string ALGOD_API_ADDR_TESTNET = "https://testnet-algorand.api.purestake.io/ps2";
string ALGOD_API_TOKEN_TESTNET = "B3SU4KcVKi94Jap2VXkK83xx38bsv95K5UZm2lab";
AlgodApi algodApiInstances = new AlgodApi(ALGOD_API_ADDR_TESTNET, ALGOD_API_TOKEN_TESTNET);
var c = address.Text;
var accountInfo = algodApiInstances.AccountInformation(c.ToString());
balance.Text = $"{accountInfo.Amount}";
await DisplayAlert("Account Address", $"MicroAlgos: {accountInfo.Amount}", "OK");
}
//Button Event Handler to Create Account Details (Address and Key)
private void Button2_Clicked(object sender, EventArgs e)
{
Account account = new Account();
AccountClass accountClass = new AccountClass()
{
Address = address.Text = account.Address.ToString(),
Key = key.Text = (account.ToMnemonic())
};
}
//How to Restore Accounts
public async Task<Account[]> RestoreAccounts()
{
Account[] accounts = new Account[3];
string mnemonic1 = "";
string mnemonic2 = "";
string mnemonic3 = "";
try
{
//Using Xamarin Esentials for Secure Storage
mnemonic1 = await SecureStorage.GetAsync(StorageAccountName1);
mnemonic2 = await SecureStorage.GetAsync(StorageAccountName2);
mnemonic3 = await SecureStorage.GetAsync(StorageAccountName3);
}
catch (Exception ex)
{
Debug.WriteLine("Error: " + ex.Message);
// Possible that device doesn't support secure storage on device.
}
// restore accounts
accounts[0] = new Account(mnemonic1);
accounts[1] = new Account(mnemonic2);
accounts[2] = new Account(mnemonic3);
return accounts;
}
}
}
5. Application Startup Page
In this closing phase, we will set up the page our application will navigate to after we start it up. In this scenario, we will use the AccountPage as our startup page. We can do that by following the code in the image below:
Figure 1-6
Add the following code to change the startup page:
Figure 1-7
6. Fund Account
To fund your account after generating your account address, visit the Algorand Bank TestNet dispenser to fund your account:
https://bank.testnet.algorand.network/
Figure 1-8
7. Deploy Application
Select the Startup Project and Proceed to deploy the application on either your Emulator, iOS or Android Device.
8. Sample Image of Application
Figure 1-9
Figure 1-10
Figure 1-11
Check the Account Balance after funding the account using the Algorand Dispenser shown above in Figure 1-8 and check the balance:
9. Complete Project
Source code can be found on GitHub: https://github.com/KusuConsult-NG/MobileAlgorand