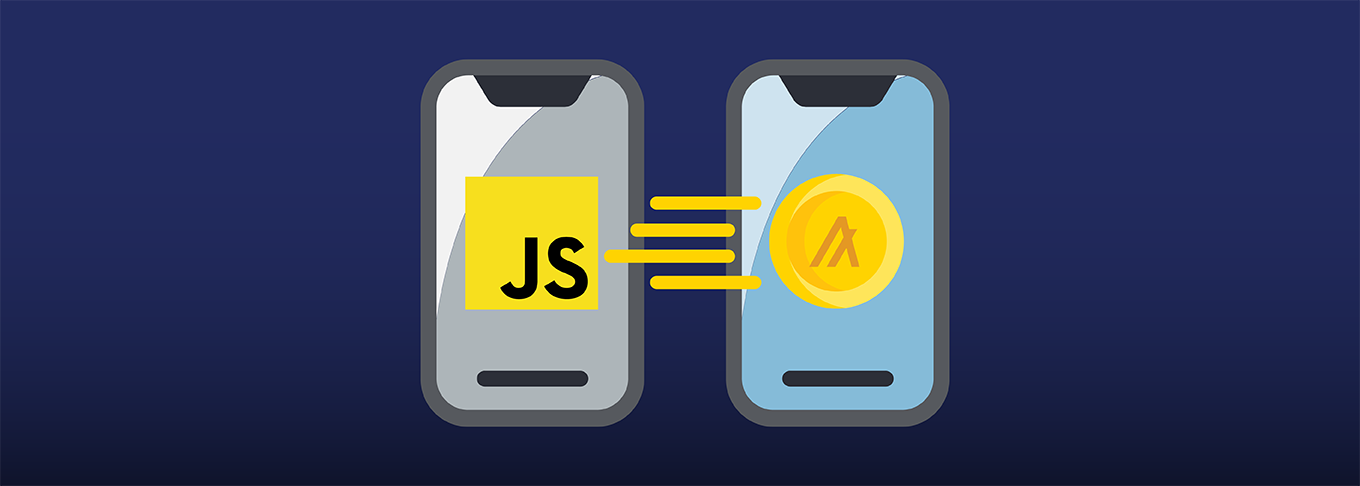
Creating a JavaScript Transaction with the PureStake API
Creating a transaction and sending it in to the network programmatically are foundational tasks for working with a blockchain. Using the PureStake API allows a developer full access to the complete Algorand MainNet/Testnet ledgers without running any infrastructure, but does require a few minor changes to the code.
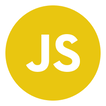
Requirements
- The JavaScript SDK: Download Here
- A PureStake Account: See Tutorial
- A Funded TestNet Account: See Tutorial
Background
- The PureStake API Service https://developer.purestake.io/
- About the PureStake API: https://www.purestake.com/technology/algorand-api/
- Code Samples: https://developer.purestake.io/code-samples
- API Examples: https://github.com/PureStake/api-examples
Steps
1. Set Configuration Values for the PureStake API
First, you’ll need to set the default configuration values for PureStake’s API. This process varies from the Algorand-published examples in that you’ll need to set the token as an object.
To do that, enter the following:
const algosdk = require('algosdk');
const baseServer = 'https://testnet-algorand.api.purestake.io/ps2';
const port = '';
const token = {
'X-API-Key': 'YOUR API KEY HERE'
}
2. Create the Client
To instantiate the client, enter:
const algodClient = new algosdk.Algodv2(token, baseServer, port);
3. Recover Account
Now you’ll need to recover your account using your mnemonic.
Important Note: Mnemonics control your account. Never share them or store insecurely. The code below is for demonstration purposes only.
const mnemonic = 'YOUR MNEMONIC HERE';
const recoveredAccount = algosdk.mnemonicToSecretKey(mnemonic);
4. Create a Transaction
Create an async function. Then fetch the transaction parameters from the blockchain and build the transaction - setting the amount and destination address - then sign it and retrieve the unique transaction ID.
(async() => {
let params = await algodClient.getTransactionParams().do();
let txn = {
"from": recoveredAccount.addr,
"to": "UUOB7ZC2IEE4A7JO4WY4TXKXWDFNATM43TL73IZRAFIFFOE6ORPKC7Q62E",
"amount": 10,
"fee": params.fee,
"firstRound": params.firstRound,
"lastRound": params.lastRound,
"genesisID": params.genesisID,
"genesisHash": params.genesisHash,
"note": new Uint8Array(0),
};
let signedTxn = algosdk.signTransaction(txn, recoveredAccount.sk);
})().catch(e => {
console.log(e);
});
5. Add a Verification Function
You will want to verify that your transaction was successfully written to the blockchain. Add the following function. We will add a call to this function in the following step.
// Function Borrowed from Algorand Inc.
const waitForConfirmation = async function (algodClient, txId) {
let lastround = (await algodClient.status().do())['last-round'];
while (true) {
const pendingInfo = await algodClient.pendingTransactionInformation(txId).do();
if (pendingInfo['confirmed-round'] !== null && pendingInfo['confirmed-round'] > 0) {
//Got the completed Transaction
console.log('Transaction confirmed in round ' + pendingInfo['confirmed-round']);
break;
}
lastround++;
await algodClient.statusAfterBlock(lastround).do();
}
};
6. Add Transaction Post and Verification
Add a call to send the transaction to the blockchain, and then a call to our new verification, to the async function, at the bottom.
const signedTxn = algosdk.signTransaction(txn, recoveredAccount.sk);
const sendTx = await algodClient.sendRawTransaction(signedTxn.blob).do();
console.log("Transaction sent with ID " + sendTx.txId);
waitForConfirmation(algodClient, sendTx.txId);
})().catch(e => {
console.log(e);
});
7. Execute and Verify transaction
Save and run. The async function is auto-invoked, so you should see an output that is similar to the following:
Transaction sent with ID YXVXP6M3CEC65JWZFK34TNJBPMO2EHUDDXCOB3K6M47ZBAIPX74A
Transaction confirmed in round 10461073
8. Run All of the Code Together
If you’d rather just drop in the code in its entirety, copy below. You’ll need to enter your API key, your mnemonic, and some other specifics in order for it to function properly.
const algosdk = require('algosdk');
const baseServer = 'https://testnet-algorand.api.purestake.io/ps2';
const port = '';
const token = {
'X-API-Key': 'YOUR API KEY HERE'
}
const algodClient = new algosdk.Algodv2(token, baseServer, port);
const mnemonic = 'YOUR MNEMONIC HERE';
const recoveredAccount = algosdk.mnemonicToSecretKey(mnemonic);
// Function Borrowed from Algorand Inc.
const waitForConfirmation = async function (algodClient, txId) {
let lastround = (await algodClient.status().do())['last-round'];
while (true) {
const pendingInfo = await algodClient.pendingTransactionInformation(txId).do();
if (pendingInfo['confirmed-round'] !== null && pendingInfo['confirmed-round'] > 0) {
//Got the completed Transaction
console.log('Transaction confirmed in round ' + pendingInfo['confirmed-round']);
break;
}
lastround++;
await algodClient.statusAfterBlock(lastround).do();
}
};
(async() => {
let params = await algodClient.getTransactionParams().do();
let txn = {
"from": recoveredAccount.addr,
"to": "UUOB7ZC2IEE4A7JO4WY4TXKXWDFNATM43TL73IZRAFIFFOE6ORPKC7Q62E",
"amount": 10,
"fee": params.fee,
"firstRound": params.firstRound,
"lastRound": params.lastRound,
"genesisID": params.genesisID,
"genesisHash": params.genesisHash,
"note": new Uint8Array(0),
};
const signedTxn = algosdk.signTransaction(txn, recoveredAccount.sk);
const sendTx = await algodClient.sendRawTransaction(signedTxn.blob).do();
console.log("Transaction sent with ID " + sendTx.txId);
waitForConfirmation(algodClient, sendTx.txId)
})().catch(e => {
console.log(e);
});