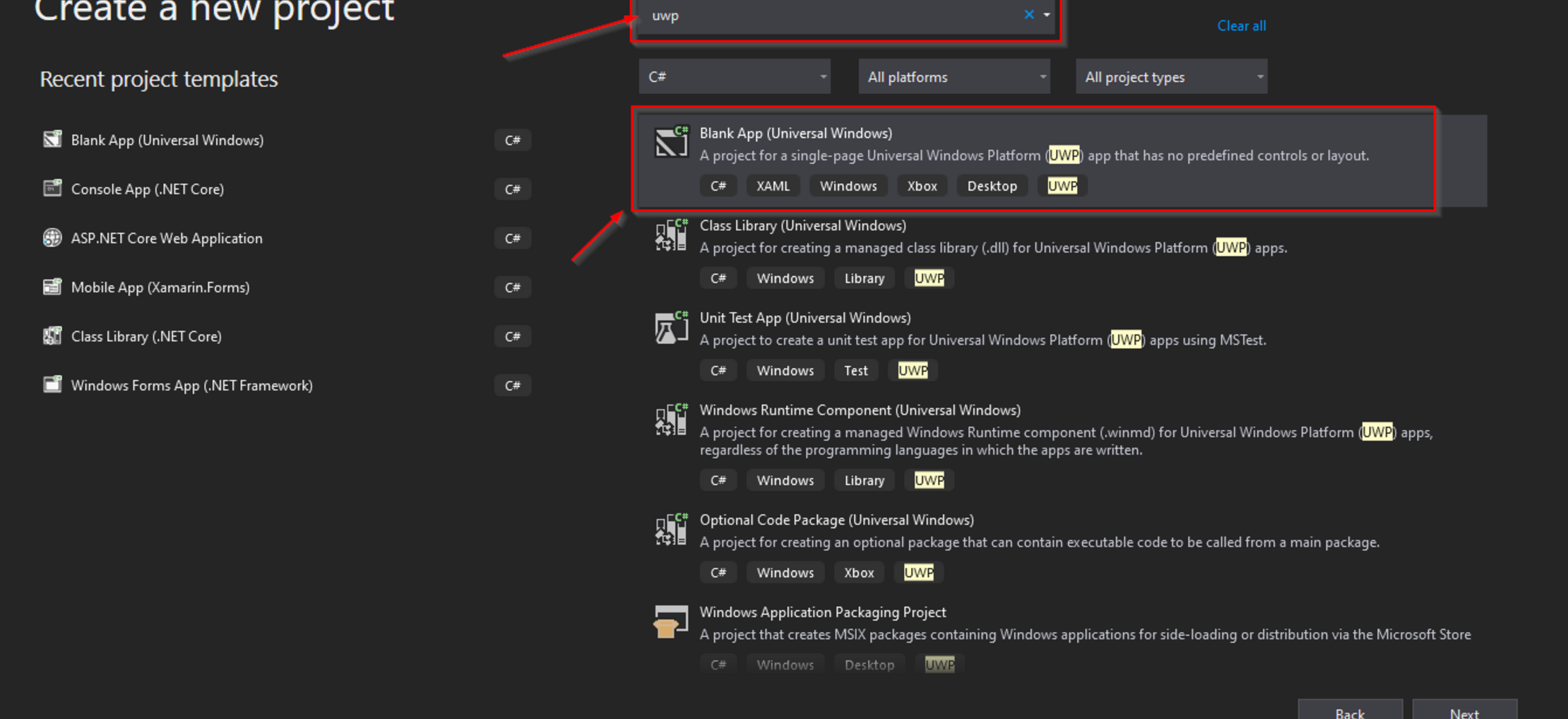
Desktop Algorand App
This tutorial teaches you how to:
- How to create Windows Applications using the C#/.NET Framework with Universal Windows Platform (UWP)
- How to install and use the Algorand Package in your application
- Interact between your application and the Algorand network to perform transactions
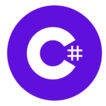
Requirements
- Algorand .NET SDK Package is required. Use the NuGet manger or the package manager console with the following command “Install-Package Algorand”
- Visual Studio Community 2019 is required. Can be downloaded from the link. https://visualstudio.microsoft.com/downloads/
Background
This is a Windows Application through which one can generate account addresses and mnemonic keys, fund the account and also check the account details. This is built using the C#/.NET framework to communicate with the Algorand Blockchain.
Steps
1. Create a UWP Application
In this tutorial, Visual Studio IDE was used to perform the tasks described above.
Create a Desktop Application (UWP) as shown in Figure 1-1 below:
Figure 1-1
Search for UWP and select the Blank App (Universal Windows) as shown in the image and click on create.
Name your project as AppUWPAlgorand and select the target versions or use the default target version options.
See Figure 1-2 below:
Figure 1-2
2. Install the Algorand Package
To Install the Algorand Package, right click on the project file and click on the “Manage NuGet Packages” as shown below to install the package.
Figure 2-1
Figure 2-2
3. Design Form Controls
Use the buttons and text fields from the Toolbox option as shown below to add the textboxes used to display account address and mnemonoic key.
Proceed to add the following code snippets in the MainPage.xaml.cs
as shown:
Focus on the TextBox additions in the Grid.
<Page
x:Class="AppUWPAlgorand.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:AppUWPAlgorand"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid>
<TextBox HorizontalAlignment="Center" Margin="549,422,0,0" Text="{x:Bind Path=ViewModel.AccountAddress}" TextWrapping="Wrap" VerticalAlignment="Top" TextChanged="TextBox_TextChanged"/>
<TextBox HorizontalAlignment="Center" Margin="549,500,0,0" Text="{x:Bind Path=ViewModel.MnemonicKey}" TextWrapping="Wrap" VerticalAlignment="Top" TextChanged="TextBox_TextChanged_1"/>
</Grid>
</Page>
We can see the two textboxes added, edit the textboxes as shown in the code above.
4. Code Behind Functionalities
Proceed to add the following code snippets in the MainPage.xaml.cs
as shown to generate an account address and a mnemonic key.
public MainPage()
{
this.InitializeComponent();
this.ViewModel = new AccountViewModel();
}
public AccountViewModel ViewModel;
public class AccountViewModel
{
public AccountViewModel()
{
Account account = new Account();
var address = account.Address.ToString();
var key = account.ToMnemonic();
this.AccountAddress = address;
this.MnemonicKey = key;
}
public string AccountAddress;
public string MnemonicKey;
}
Add the following namespaces below to the already existing ones:
using Algorand;
using Algorand.V2;
using Algorand.Client;
using Algorand.V2.Model;
using Account = Algorand.Account;
using Windows.UI.Popups;
5. Debug/Run the application
Debug/Run the Application to generate the account address and mnemonic key as shown in Figure 5-1 below.
Note: Do not share you Key with the public as the one used for this tutorial is for testing purposes.
The application will work and look like the image below:
Figure 5-1
6. Configure your code to check the account address balance
Inside of the MainPage.xaml.cs
proceed to add a button as shown below:
Note: Comment out the TextBoxes earlier added so as not to generate another account address/key as shown below:
Proceed to add a button and navigate back to the MainPage.xaml.cs
file.
<Grid>
<Button Content="Get Account Balance" VerticalAlignment="Center" HorizontalAlignment="Center" Click="Button_Click_1"/>
<!--<TextBox HorizontalAlignment="Center" Margin="0,430,0,0" Text="{x:Bind Path=ViewModel.AccountAddress}" TextWrapping="Wrap" VerticalAlignment="Top" TextChanged="TextBox_TextChanged"/>
<TextBox HorizontalAlignment="Center" Margin="0,500,0,0" Text="{x:Bind Path=ViewModel.MnemonicKey}" TextWrapping="Wrap" VerticalAlignment="Top" TextChanged="TextBox_TextChanged_1" RenderTransformOrigin="0.219,0.438"/>-->
</Grid>
MainPage.xaml.cs
Add the following code snippets below in the Button Event Hanlder method to check account balance details.
private async void Button_Click_1(object sender, RoutedEventArgs e)
{
string ALGOD_API_ADDR = "https://testnet-algorand.api.purestake.io/ps2";
string ALGOD_API_TOKEN = "B3SU4KcVKi94Jap2VXkK83xx38bsv95K5UZm2lab";
AlgodApi algodApiInstance = new AlgodApi(ALGOD_API_ADDR, ALGOD_API_TOKEN);
var accountInfo = algodApiInstance.AccountInformation("VYFF3MXAXWGHNIAEUVINZZHEW2MH5HKTXEACXUTZ3HHADIBPT5ATJGR6K4");
var dialog = new MessageDialog(string.Format("Account Balance: " + accountInfo.Amount + " MicroAlgos"));
await dialog.ShowAsync();
}
7. Fund Account Address
To fund the account address, use the Algorand TestNet dispenser to dispense funds into the account: https://bank.testnet.algorand.network/
Figure 7-1
8. Debug/Run Application
Figure 8-1
Click on the button to display the account address balance:
9. Source Code Sample
The complete project sample can be found in the link:
https://github.com/KusuConsult-NG/AppUWPAlgorand
10. Video Tutorial
The complete video tutorial of the application can be found here: