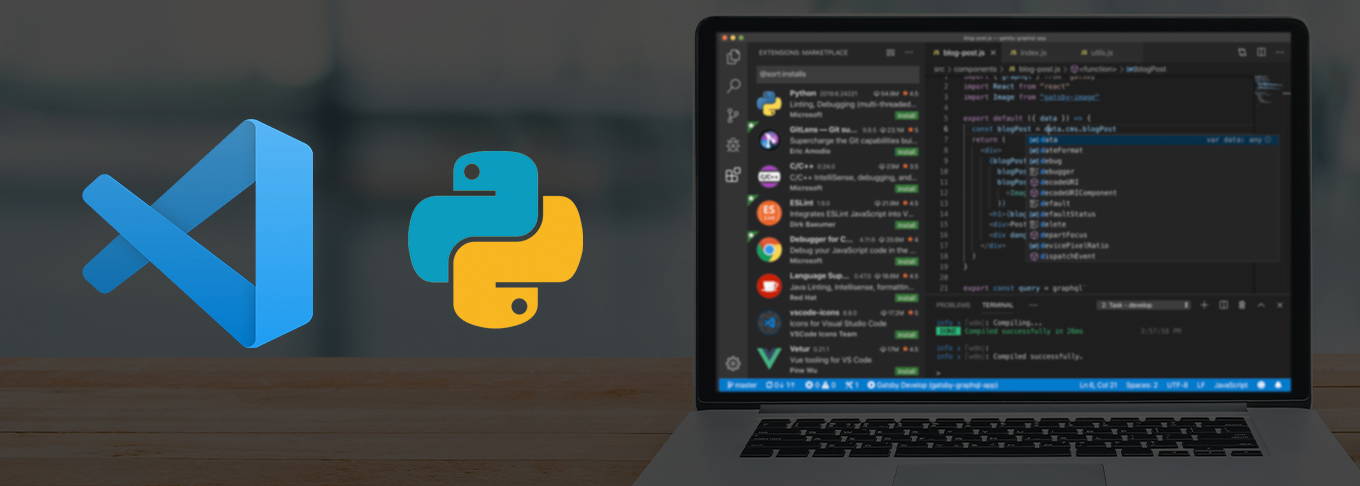
Using VS Code with Python
Being able to debug is a great tool when learning new programming content. This tutorial will facilitate how to debug using Visual Studio (VS) Code, which can be used with all the code samples on the Algorand Developer Docs Portal. Other debuggers can also be used. If you are new to debugging, VS Code is the most popular tool. It supports many programming languages, is quick to load and has a wealth of documentation and extensions.
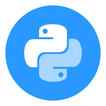
Requirements
- The sample in this tutorial requires Python SDK Installation.
Background
Visual Studio Code is a lightweight but powerful source code editor that runs on your desktop and is available for Windows, macOS, and Linux. It comes with built-in support for JavaScript, TypeScript and Node.js and has a rich ecosystem of extensions for other languages. VS Code can take on most of the tasks of an Integrated Development Environment (IDE) with the right configuration and plugin library extension. VS Code is open source.
Extensions are available in most development languages for VS Code. Specific install instructions are in Step 2 below. For general information see: Java, JavaScript, Go, Python and C#. See extensions for VS Code languages here.
For documentation, several other extensions are available including Grammarly and MarkDown (MD).
This tutorial walks through Python examples.
Steps
1. Download VS Code
Download VS Code from this site https://code.visualstudio.com/Download
2. Install Extensions
Open VS Code. Go to the Extensions view.
Filter the extensions list by typing your programming language of choice, such as Python, and you will see all related extensions. Install Microsoft’s Python Extension
Figure 2-1 Python Extension Marketplace Search
3. Debug with VS Code
For general information on setting up debugging in VS Code see this:
https://code.visualstudio.com/docs/editor/debugging
Optionally, for Keyboard shortcuts and Keymap extensions, which match other editors, see this:
https://code.visualstudio.com/docs/getstarted/keybindings
4. Debugging Python with VS Code sample
In this step, we will show how to create a debug session for Python using VS Code. The steps are similar for all languages. Here is a sample Python file to test with. This code generates an Account and retrieves the mnemonic. Name this file GenerateAlgorandKeypair.py
from algosdk import account, mnemonic
private_key, address = account.generate_account()
print("My address: {}".format(address))
print("My passphrase: {}".format(mnemonic.from_private_key(private_key)))
Figure 4-1 Press Run Debug Python (F5)
A) Select the Debug icon on the left
B) Set a breakpoint in the code. To set a breakpoint, simply click on the left margin, sometimes referred to as the gutter, on the line to break.
C) See the breakpoints set in the debug pane.
D) Press Run and Debug and you should hit your breakpoint. If you do not see a Run and Debug button, this means you already have a launch.json. Open it to see the settings, it is located in the .vscode folder in your code folder. Each configuration should appear in the Debug and Run drop-down menu.
E) You may be prompted for the debug configuration. If so, select Python.
F) Optionally, to customize Run and Debug, click on “Create a launch.json”. Launch.json is the setting file for the debugger.
Your breakpoint should be hit, and the program execution paused on the line of your breakpoint. It is highlighted.
Figure 4-2 Debugging.
We are now debugging! See Figure 4-2 above.
A) Local Variables
B) Hover over a variable with the cursor. See the Data Tip with the contents
C) Navigate by selecting run (to next breakpoint), step over, step into, step out of or stop
Learn More