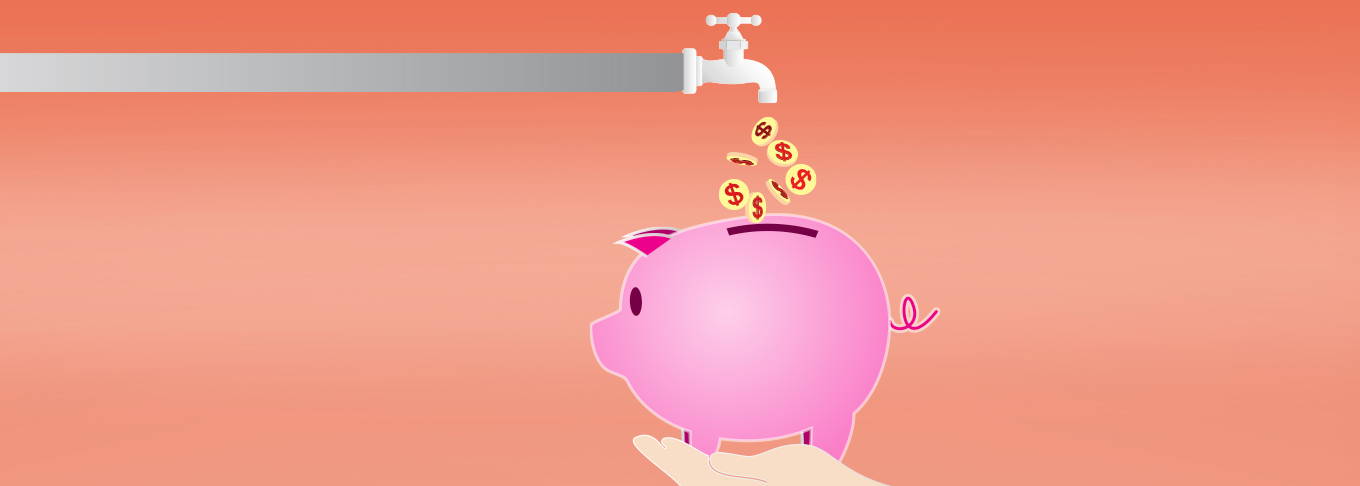
Create an Account on TestNet with Python
Generate a new standalone account, fund it with the TestNet faucet, and check your balance using the Python SDK.
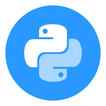
Requirements
Background
To send any type of transaction, you must first have an account. This tutorial focuses on generating a Standalone Account. To learn more about Accounts on Algorand visit the Accounts Overview page in the docs.
Steps
1. Generate an Algorand Key Pair.
Import the account
module from the Python sdk.
>>> from algosdk import account
Generate a public/private keypair with the generate_account
function.
>>> private_key, public_address = account.generate_account()
Print the private key an Algorand address so you can see what they look like.
>>> print("Base64 Private Key: {}\nPublic Algorand Address: {}\n".format(private_key, public_address))
Base64 Private Key: cvc2Zgq8GVTtCAR9PAC8O6rLn7s39QdOKU3ldvLK6zffxHg1oanbrGbU3kL6w0NeB4KO2ChJHaaDf25Zb1OqZw==
Public Algorand Address: 37CHQNNBVHN2YZWU3ZBPVQ2DLYDYFDWYFBER3JUDP5XFS32TVJT3ZEXNE4
The private key is the base64 representation and the Algorand address is the base32 checksummed version of the public key.
2. Retrieve the Private Key Mnemonic
Transform this key into a mnemonic that you can save for later use. To do this, first import the mnemonic
module. Then export the private key as a mnemonic.
>>> from algosdk import mnemonic
>>> mnemonic.from_private_key(private_key)
'unusual swift credit scheme cricket fence electric advice moral abstract task photo nuclear tree saddle vivid science pioneer pledge hour top verify satisfy ability palace'
Note that the mnemonic will be different set of 25 words each time you run this tutorial. Because we are on TestNet and demonstrating an example, we are openly sharing this private key. You should never do this in real life; especially when your private key represents an account with real-value assets.
With this mnemonic, anyone can sign spend transactions from the corresponding account.
3. Send Algos to the New Account
Let’s send Algos to the new account by visiting the TestNet faucet linked here.
Enter the Algorand Public Address in the textbox, complete the recaptcha, and click “Dispense”. If you receive a 200 status code, your account should now be funded with 100 Algos.
4. Completed Code
The following code generates 3 accounts. It is sometimes useful to have multiple accounts. Three accounts will be needed for ASA tutorials for example. Be sure to fund each generated account using the TestNet Dispenser as described above.
import json
from algosdk import account, mnemonic
acct = account.generate_account()
address1 = acct[1]
print("Account 1")
print(address1)
mnemonic1 = mnemonic.from_private_key(acct[0])
print("Account 2")
acct = account.generate_account()
address2 = acct[1]
print(address2)
mnemonic2 = mnemonic.from_private_key(acct[0])
print("Account 3")
acct = account.generate_account()
address3 = acct[1]
print(address3)
mnemonic3 = mnemonic.from_private_key(acct[0])
print ("")
print("Copy off accounts above and add TestNet Algo funds using the TestNet Dispenser at https://bank.testnet.algorand.network/")
print("copy off the following mnemonic code for use later")
print("")
print("mnemonic1 = \"{}\"".format(mnemonic1))
print("mnemonic2 = \"{}\"".format(mnemonic2))
print("mnemonic3 = \"{}\"".format(mnemonic3))
# terminal output should look similar to this
# Account 1
# KECA5Z2ZILJOH2ZG7OPKJ5KMFXP5XBAOC7H36TLPJOQI3KB5UIYUD5XTZU
# Account 2
# DWQ4IA7EK5BKHSPNCJBA5SOVU66TKGUCCGO2SHQCI5UB2JAO3G2GWXMGPA
# Account 3
# TABDMZ2EUTNOR3S74SJWW37DLHE7BDGS5XB5JPLFQ2VQVJOE2DXKX722VU
# Copy off accounts above and add TestNet Algo funds using the TestNet Dispenser at
# https: // bank.testnet.algorand.network/
# copy off the following mnemonic code for use later
# mnemonic1 = "consider round clerk soldier hurt dynamic floor video output spoon deliver virtual zoo inspire rubber doll nose warfare improve abstract recall choice size above actor"
# mnemonic2 = "boil explain enlist adapt science hub universe knife ghost scheme lazy payment must gas coconut forget goddess author filter civil tumble antique delay absorb lend"
# mnemonic3 = "place elbow thumb bid taste strong sting tube swarm comic wave dinosaur congress sword zebra need proud primary brief rotate story pilot garbage abstract black"
5. Check Your Balance
You can check your balance with any block explorer connected to TestNet.
You can also connect to a node through the Python algod client. Make sure to first retrieve an IP address and access token through one of the methods described in the Workspace Setup.
Once you have an address and token. Instantiate a client and call the account_info method to check the account’s balance.
>>> from algosdk.v2client import algod
>>> import json
>>> ip_address = <algod-address>
>>> token = <algod-token>
>>> client = algod.AlgodClient(token, ip_address)
>>> account_info = client.account_info('37CHQNNBVHN2YZWU3ZBPVQ2DLYDYFDWYFBER3JUDP5XFS32TVJT3ZEXNE4')
>>> print(json.dumps(account_info, indent=4))
{
"round": 4995033,
"address": "37CHQNNBVHN2YZWU3ZBPVQ2DLYDYFDWYFBER3JUDP5XFS32TVJT3ZEXNE4",
"amount": 100000000,
"pendingrewards": 0,
"amountwithoutpendingrewards": 100000000,
"rewards": 0,
"status": "Offline"
}
>>> account_balance = account_info.get('amount')
>>> print('Account balance: {} microAlgos'.format(account_balance))
Account balance: 100000000 microAlgos