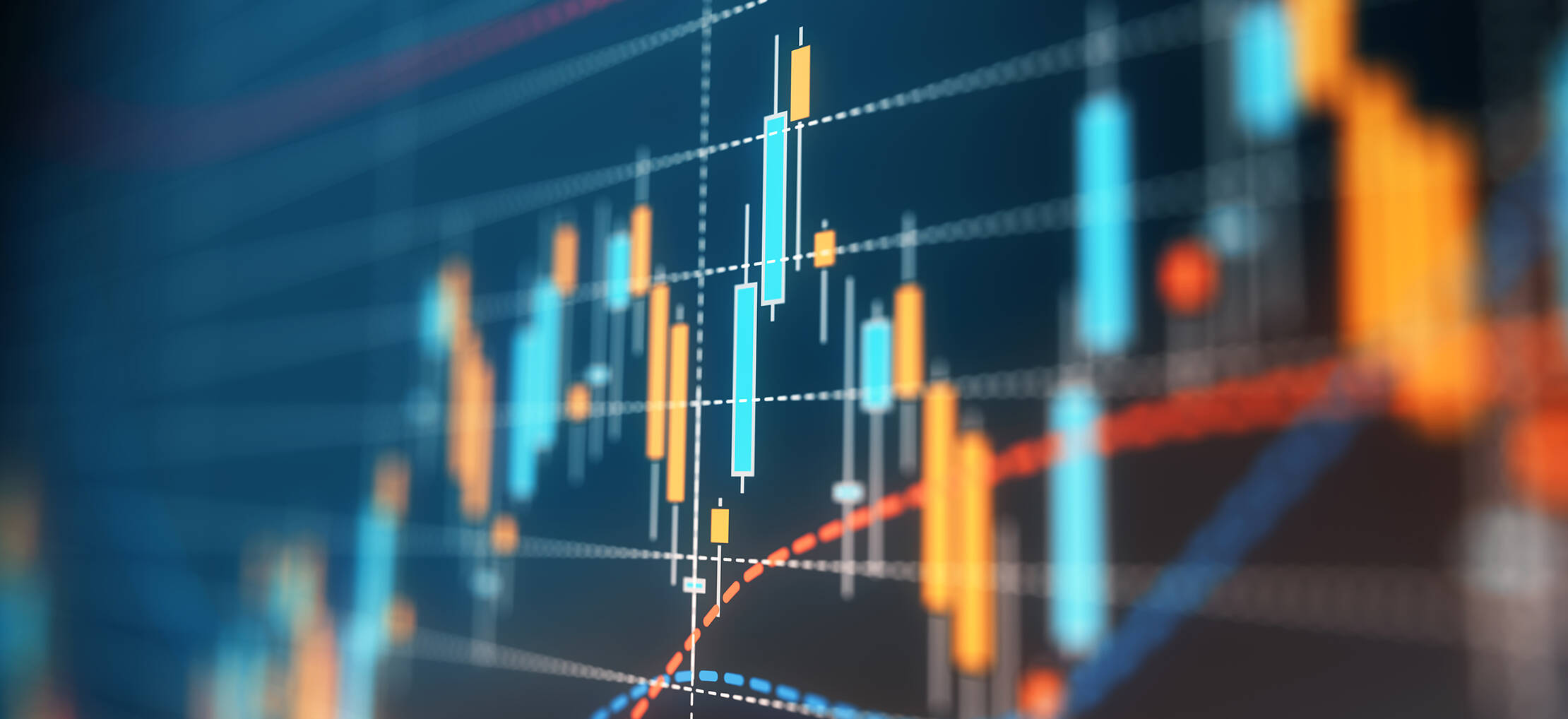
LimitOrder Contract with Java
This tutorial is intended to help you call a Limit Order Contract using Java. Templates are prebuilt TEAL programs that allow parameters to be injected into them from the SDKs that configure the contract. In this example, we are going to instantiate the LimitOrder Template and show how it can be used with a transaction.

Requirements
- Algorand Java SDK
- Access to an Algorand node
- an Algorand asset to use in the contract
Background
Algorand provides many templates for Smart Contract implementation in the SDKs. The LimitOrder Contract is just one of the templates and is described in the reference documentation. Limit Order Contracts are contract accounts that can disburse Algos for Algorand Assets in a defined ratio. Additionally, a minimum withdrawal amount can be set. If the funds are not claimed after a certain period of time, the original owner can reclaim the remaining funds.
Steps
1. Get Asset Owner and the Contract Owner Accounts
The Limit Order template can be instantiated with a set of predefined parameters that configure the Limit Order contract. These parameters should not be confused with Transaction parameters that are passed into the contract when using the Limit Order. These parameters configure how the Limit Order will function:
TMPL_ASSET
: Integer ID of the assetTMPL_SWAPN
: Numerator of the exchange rate (TMPL_SWAPN assets per TMPL_SWAPD microAlgos, or better)TMPL_SWAPD
: Denominator of the exchange rate (TMPL_SWAPN assets per TMPL_SWAPD microAlgos, or better)TMPL_TIMEOUT
: The round after which all of the algos in this contract may be closed back to TMPL_OWNTMPL_OWN
: The recipient of the asset (if the order is filled), or of the contract’s algo balance (after TMPL_TIMEOUT)TMPL_FEE
: The maximum fee used in any transaction spending out of this contractTMPL_MINTRD
: The minimum number of microAlgos that may be spent out of this contract as part of a trade
So for example, If you want to specify that the contact will approve a transaction where it is willing to spend 3000 microAlgos for 1 Asset with id of TMPL_ASSET
, you would set the TMPL_SWAPN
parameter to 1 and the TMPL_SWAPD
to 3000. The TMPL_MINTRD
should be set to 2999 in this example as it must be less than the amount of microAlgos for the one asset.
For this tutorial, we first need to define, the owner of the contract, and the owner of the asset with asset id = TMPL_ASSET
.
package com.algorand.algosdk.teal;
import java.math.BigInteger;
import com.algorand.algosdk.account.Account;
import com.algorand.algosdk.algod.client.AlgodClient;
import com.algorand.algosdk.algod.client.api.AlgodApi;
import com.algorand.algosdk.algod.client.auth.ApiKeyAuth;
import com.algorand.algosdk.algod.client.model.TransactionID;
import com.algorand.algosdk.algod.client.model.TransactionParams;
import com.algorand.algosdk.crypto.Address;
import com.algorand.algosdk.crypto.Digest;
import com.algorand.algosdk.templates.ContractTemplate;
import com.algorand.algosdk.templates.LimitOrder;
/**
* Sign and Submit a transaction example using LimitOrder
*
*/
public class SubmitLimitTransaction
{
public static void main(String args[]) throws Exception {
// Setup conection parameters
final String ALGOD_API_ADDR = "http://<your-algod-host>:<your-algod-port>";
final String ALGOD_API_TOKEN = "<your-api-token>";
// Instaniate the algod wrapper
AlgodClient client = (AlgodClient) new AlgodClient().setBasePath(ALGOD_API_ADDR);
ApiKeyAuth api_key = (ApiKeyAuth) client.getAuthentication("api_key");
api_key.setApiKey(ALGOD_API_TOKEN);
AlgodApi algodApiInstance = new AlgodApi(client);
// Owner is the owner of the contract
Address owner = new Address("AJNNFQN7DSR7QEY766V7JDG35OPM53ZSNF7CU264AWOOUGSZBMLMSKCRIU" );
// Recover accounts used in example
// Account 1 is the asset owner
// Used later in the example
String account1_mnemonic = "portion never forward pill lunch organ biology" +
" weird catch curve isolate plug innocent skin grunt" +
" bounce clown mercy hole eagle soul chunk type absorb trim";
Account assetOwner = new Account(account1_mnemonic);
}
}
2. Create Limit Order Template
The template can now be created with the correct ratio for assets to microAlgos.
package com.algorand.algosdk.teal;
import java.math.BigInteger;
import com.algorand.algosdk.account.Account;
import com.algorand.algosdk.algod.client.AlgodClient;
import com.algorand.algosdk.algod.client.api.AlgodApi;
import com.algorand.algosdk.algod.client.auth.ApiKeyAuth;
import com.algorand.algosdk.algod.client.model.TransactionID;
import com.algorand.algosdk.algod.client.model.TransactionParams;
import com.algorand.algosdk.crypto.Address;
import com.algorand.algosdk.crypto.Digest;
import com.algorand.algosdk.templates.ContractTemplate;
import com.algorand.algosdk.templates.LimitOrder;
/**
* Sign and Submit a transaction example using LimitOrder
*
*/
public class SubmitLimitTransaction
{
public static void main(String args[]) throws Exception {
// Setup conection parameters
final String ALGOD_API_ADDR = "http://<your-algod-host>:<your-algod-port>";
final String ALGOD_API_TOKEN = "<your-api-token>";
// Instaniate the algod wrapper
AlgodClient client = (AlgodClient) new AlgodClient().setBasePath(ALGOD_API_ADDR);
ApiKeyAuth api_key = (ApiKeyAuth) client.getAuthentication("api_key");
api_key.setApiKey(ALGOD_API_TOKEN);
AlgodApi algodApiInstance = new AlgodApi(client);
// Owner is the owner of the contract
Address owner = new Address("AJNNFQN7DSR7QEY766V7JDG35OPM53ZSNF7CU264AWOOUGSZBMLMSKCRIU" );
// Recover accounts used in example
// Account 1 is the asset owner
// Used later in the example
String account1_mnemonic = "portion never forward pill lunch organ biology" +
" weird catch curve isolate plug innocent skin grunt" +
" bounce clown mercy hole eagle soul chunk type absorb trim";
Account assetOwner = new Account(account1_mnemonic);
// Inputs
// Limit contract should be two receivers in an
// ratn/ratd ratio of assetid to microalgos
// ratn is the number of assets
// ratd is the number of microAlgos
int expiryRound = 5000000;
int maxFee = 2000;
int minTrade = 2999;
Integer ratn = 1;
Integer ratd = 3000;
// assetID is the asset id number
// of the asset to be traded
int assetID = 316084;
// Instantiate the templates
ContractTemplate limit = LimitOrder.MakeLimitOrder(owner, assetID, ratn, ratd, expiryRound, minTrade, maxFee);
// At this point the contract can be funded
// The program bytes can be saved to be used later
// or in a differnt application
System.out.println("Contract address: " + limit.address);
byte[] contractProgram = limit.program;
}
}
At this point, the Limit Order contract account must be funded before any transactions can be issued against it. Use the dispenser to do this now. Also, note that program bytes can be saved to use at a later time or in another application at this point.
Learn More
- Add Funds using Dispenser
- Smart Contracts - Contract Accounts
3. Create and Submit Transaction against Contract
The Limit Order contract template offers a helper method to create the proper transactions against the contract called MakeSwapAssetsTransaction
. This function takes an assetAmount
and a microAlgoAmount
for the total transaction and creates two transactions, one for each receiver and then groups these two transactions into an atomic transfer. The first transaction is signed with the program logic and the second transaction is signed with the secret key of the asset owner. The bytes of the grouped transactions to submit are returned. These bytes can then be submitted to the blockchain.
package com.algorand.algosdk.teal;
import java.math.BigInteger;
import com.algorand.algosdk.account.Account;
import com.algorand.algosdk.algod.client.AlgodClient;
import com.algorand.algosdk.algod.client.api.AlgodApi;
import com.algorand.algosdk.algod.client.auth.ApiKeyAuth;
import com.algorand.algosdk.algod.client.model.TransactionID;
import com.algorand.algosdk.algod.client.model.TransactionParams;
import com.algorand.algosdk.crypto.Address;
import com.algorand.algosdk.crypto.Digest;
import com.algorand.algosdk.templates.ContractTemplate;
import com.algorand.algosdk.templates.LimitOrder;
/**
* Sign and Submit a transaction example using LimitOrder
*
*/
public class SubmitLimitTransaction
{
public static void main(String args[]) throws Exception {
// Setup conection parameters
final String ALGOD_API_ADDR = "http://<your-algod-host>:<your-algod-port>";
final String ALGOD_API_TOKEN = "<your-api-token>";
// Instaniate the algod wrapper
AlgodClient client = (AlgodClient) new AlgodClient().setBasePath(ALGOD_API_ADDR);
ApiKeyAuth api_key = (ApiKeyAuth) client.getAuthentication("api_key");
api_key.setApiKey(ALGOD_API_TOKEN);
AlgodApi algodApiInstance = new AlgodApi(client);
// Owner is the owner of the contract
Address owner = new Address("AJNNFQN7DSR7QEY766V7JDG35OPM53ZSNF7CU264AWOOUGSZBMLMSKCRIU" );
// Recover accounts used in example
// Account 1 is the asset owner
// Used later in the example
String account1_mnemonic = "portion never forward pill lunch organ biology" +
" weird catch curve isolate plug innocent skin grunt" +
" bounce clown mercy hole eagle soul chunk type absorb trim";
Account assetOwner = new Account(account1_mnemonic);
// Inputs
// Limit contract should be two receivers in an
// ratn/ratd ratio of assetid to microalgos
// ratn is the number of assets
// ratd is the number of microAlgos
int expiryRound = 5000000;
int maxFee = 2000;
int minTrade = 2999;
Integer ratn = 1;
Integer ratd = 3000;
// assetID is the asset id number
// of the asset to be traded
int assetID = 316084;
// Instantiate the templates
ContractTemplate limit = LimitOrder.MakeLimitOrder(owner, assetID, ratn, ratd, expiryRound, minTrade, maxFee);
// At this point the contract can be funded
// The program bytes can be saved to be used later
// or in a differnt application
System.out.println("Contract address: " + limit.address);
byte[] contractProgram = limit.program;
int assetAmount = 1;
int microAlgoAmount = 3000;
// set to get minimum fee
int feePerByte = 0;
TransactionParams params = algodApiInstance.transactionParams();
// Load the contract template and create the grouped
// transactions for the split contract
ContractTemplate loadedContract = new ContractTemplate(contractProgram);
byte[] transactions = LimitOrder.MakeSwapAssetsTransaction(
loadedContract,
assetAmount,
microAlgoAmount,
assetOwner,
params.getLastRound().intValue(),
params.getLastRound().add(BigInteger.valueOf(500)).intValue(),
new Digest(params.getGenesishashb64()), feePerByte);
// Submit the transactions
try {
TransactionID id = algodApiInstance.rawTransaction(transactions);
System.out.println("Successfully sent tx with id: " + id);
} catch (Exception e) {
// This is generally expected, but should give us an informative error message.
System.err.println("Exception when calling algod#rawTransaction: " + e);
}
}
}
Learn More
- Atomic Transfers